Baze
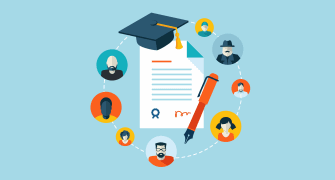
Master ADO.NET and LINQ Challenge
Test your knowledge and skills in ADO.NET and LINQ with this comprehensive quiz! Whether you're a student, a developer, or just someone looking to learn more about data management in .NET, this quiz covers essential concepts and practical applications.
You'll encounter a variety of topics, including:
- Data management techniques
- Working with SQL Server
- Linq queries and methods
- XML processing in .NET
1. Which class should you use to manage multiple tables and relationships among them?
DataSet
DataRow
DataView
DataTable
2. You want to set up a primary key on a column. Which properties on the data column must be set? (Each correct answer presents part of a complete solution. Choose three.)
MappingType
AutoIncrementSeed
AutoIncrementStep
AutoIncrement
If you want to assign a Car object to a column called CarObject, which attribute must be on the Car class to enable saving the data table to a file?
Bindable
DataObject
Serializable
FileIOPermission
You are storing custom Car objects in a data table whose DataView property displays a filtered list of Car objects, filtered on Make Of The Cars. If you change the make of one of the Car objects, you want to ensure that the filter will dynamically update. What must you do to be assured that the filter will detect the change made to your custom Car object?
Create a new Car object and assign it to the column value
Nothing, because this scenario works by default
You are storing custom Car objects in a data table that will be serialized to a file. After serializing to a binary file called cars.bin, you open the file with a binary editor and notice that XML is in the file. Which setting can you use to ensure that you will create a binary file without embedded XML?
You are storing custom Car objects in a data table that will be serialized to a file. After serializing to a binary file called cars.bin, you open the file with a binary editor and notice that XML is in the file. Which setting can you use to ensure that you will create a binary file without embedded XML?
Set the RemotingFormat property to SerializationFormat.Binary
Set the DefaultView property to SerializationFormat.Binary.
Set the Constraints property to SerializationFormat.Binary
When working with a data set that has data in it, you decide you want to store the schema, but not the data, of the data set to a file so you can share the schema with other users. Which method must you execute to store the schema?
InferXmlSchema
ReadXmlSchema
WriteXmlSchema
WriteXml
You want to create a connection to an old SQL server. Upon investigation, you discover that its version is 6.5. Which .NET provider will you use to connect to this server?
Oracle
SqlClient
Oledb
Before you can execute a command on a connection object, which method must you execute to prepare the connection?
Open
BeginTransaction
GetSchema
Close
You want to set up a secure connection between your application and SQL Server. SQL Server has a trusted certificate that is set up properly. What must you do?
Execute BeginTransaction on the command object
Add Encrypt=true to the connection string
Encrypt the CommandText property on the command object.
Close the connection before sending the command.
You want to secure the connection strings contained within your Web.config file to ensure that no one can open the file easily and see the connection information. Which tool must you use to encrypt the connection strings?
ASPNET_REGSQL.EXE
CASPOL.EXE
INSTALLUTIL.EXE
ASPNET_REGIIS.EXE
. You are going to execute a Select command to SQL Server that returns several rows of customer data. You don’t need a data table to hold the data because you will simply loop over the returned results to build a string of information that will be displayed to the user. You create and open a connection and then create the command and set its properties. Which method on the command will you execute to retrieve the results?
ExecuteScalar
Close
ExecuteReader
ExecuteNonQuery
You are calling a stored procedure in SQL Server 2008 that returns a UDT as an output parameter. This UDT, called MyCompanyType, was created by your company. The UDT has a method called GetDetails that you want to execute in your client application. What must you do to execute the method? (Each correct answer presents part of a complete solution. Choose three.)
Set the SqlDbType of the parameter to SqlDbType.Udt
Set the UdtTypeName of the parameter to MyCompanyType.
In the client application, set a reference to the assembly in which the UDT is
Call the ExecuteXmlReader method on the SqlCommand to serialize the UDT as XML.
You want to execute a SQL insert statement from your client application, so you set the CommandText property of the command object and open the connection. Which method will you execute on the command?
ExecuteScalar
ExecuteXmlReader
ExecuteReader
ExecuteNonQuery
In ADO.NET, which class can start an explicit transaction to update a SQL Server database?
SqlCommand
SqlParameter
SqlConnection
SqlException
In the System.Transaction namespace, which class can start an explicit promotable transaction to update a SQL Server database?
TransactionScope
SqlConnection
SqlTransaction
You want to store the contents of a data set to an XML file so you can work on the data while disconnected from the database server. How should you store this data?
As a SOAP file
As a DataGram file
As a WSDL file
As an XML Schema file
To which of the following types can you add an extension method? (Each correct answer presents a complete solution. Choose five.)
Class
Structure
Enum
Interface
Delegate
Module
You want to page through an element sequence, displaying ten elements at a time, until you reach the end of the sequence. Which query extension method can you use to accomplish this? (Each correct answer presents part of a complete solution. Choose two.)
Skip
Except
SelectMany
Take
You have executed the Where query extension method on your collection, and it returned IEnumerable of Car, but you want to assign this to a variable whose type is List Of Car. How can you convert the IEnumerable of Car to List Of Car?
Use CType (C# cast).
It can’t be done.
Use the ToList() query extension method.
Just make the assignment.
Given the following LINQ query: from c in cars join r in repairs on c.VIN equals r.VIN … what kind of join does this perform?
Cross join
Left outer join
Right outer join
Inner join
In a LINQ query that starts with: from o in orderItems The orderItems collection is a collection of OrderItem with properties called UnitPrice, Discount, and Quantity. You want the query to filter out OrderItem objects whose totalPrice (UnitPrice * Quantity * Discount) result is less than 100. You want to sort by totalPrice, and you want to include the total price in your select clause. Which keyword can you use to create a totalPrice result within the LINQ query so you don’t have to repeat the formula three times?
Let
On
Into
By
When working with LINQ to SQL, what is the main object that moves data to and from the database?
DataSet
SqlDataAdapter
DataContext
Entity
You want to use LINQ to SQL to run queries on a table that contains a column that stores large photos. Most of the time, you won’t need to view the photo, but occasionally you will need to see it. In the LINQ to SQL designer, which property can you set on the photo column to get the efficient loading of the data for most scenarios but still be able to retrieve the photo when needed?
Skip
Delay Loaded
Take
Auto Generated Value
Which query extension methods can you use to implement paging over a LINQ to SQL query?
Contains and Intersect
GroupBy and Last
Skip and Take
First and Last
When using a LINQ query to join two tables, you must specify how the two tables are related by using which keyword?
Let
Equals
Into
By
. You retrieved a row of data into an entity object by using a LINQ to SQL DataContext object. You haven’t made any changes to the object, but you know that someone else has modified the data row in the database table, so you rerun your query, using the same LINQ to SQL DataContext object, to retrieve the updated data. What can be said about the result of the second query?
It returns the updated data and you can use it immediately.
The changes are thrown out and you use the cached data, so you don’t see the changes.
An exception is thrown due to the difference in data
An exception is thrown because you already have the object, so you can’t re-query unless you create a new DataContext object.
. You ran a LINQ to SQL query to retrieve the products that you are going to mark as discontinued. After running the query, you looped through the returned products and set their Discontinued property to true. What must you do to ensure that the changes go back to the database?
Call the Update method on the DataContext object.
Nothing; the changes are sent when you modify the object
Call the Dispose method on the DataContext object.
Call the SubmitChanges method on the DataContext object.
Given an XML file, you want to run several queries for data in the file based on filter criteria the user will be entering for a particular purpose. Which class would be more appropriate for these queries on the file?
XmlReader
XmlDocument
Every day, you receive hundreds of XML files. You are responsible for reading the file to retrieve sales data from the file and store it into a SQL database. Which class would be more appropriate for these queries on the file?
XmlDocument
XmlReader
You have a service that receives a very large XML-based history file once a month. These files can be up to 20GB in size, and you need to retrieve the header information that contains the history date range and the customer information on which this file is based. Which class would be more appropriate to retrieve the data in these files?
XmlDocument
XmlReader
Given an XML file, you want to run several queries for data using LINQ to XML. Which class would be most appropriate for these queries on the file?
XmlDocument
XmlReader
XDocument
. In your code, you have a string variable that contains XML. Which method can you use to convert this into an XDocument class so you can run LINQ to XML queries?
Load
Constructor
WriteTo
Parse
You have an XML file you want to transform to a different form of XML, and you want to use XML literals to simplify the transformation. Which language will you use?
C#
Visual Basic
Either
You are creating a new application that will use a database that already exists. Which model will you use to create your Entity Data Model?
The Code First model
The Database First model
You have a created Customer entity but it comprises properties that are spread across two tables in the database. What should you do?
Use LINQ to SQL classes.
Use LINQ to XML.
Use the Entity Framework
You have created entity classes from your conceptual model, but you can’t add a custom method to one of the classes. Which would be the best approach to adding this method?
Create a T4 text template and the method.
Add an EntityObject Generator to your project and add the new modification to the text template
Add a new partial class to your project and add the method to this class.
Open the created entity class and add the method.
In your application, you have existing classes that represent the conceptual model. How can the Entity Framework be configured to use these classes?
You can use these classes by making the classes inherit from ObjectContext.
You can use these classes, but you must make the EDMX-generated entity classes inherit from each of your existing classes.
You can implement a POCO EDMX file.
You have created a conceptual model manually by using the Entity Framework designer. Can you use the Entity Framework designer to generate a new database from the completed conceptual model?
Yes
Nop
In your application, you want to define a function to be called in your LINQ to Entities query and executed server-side, but you don’t have permission to create objects in the database server. How can you solve this problem?
Create a model-defined function
Extend the entity class and add a method to it.
Create a stored procedure
In the Entity Framework, which is the primary object you use to query and modify data?
ObjectContext
DataContext
ObjectStateEntry
EntityObject
Adding a call to the Include extension method in a query for data is an example of what kind of loading strategy?
Lazy loading
Eager loading
Explicit loading
When working with the Entity Framework, which method do you execute to send changes back to the database?
SubmitChanges
SaveChanges
You are writing an Entity SQL query that requires you to construct a row from various data. Which function will you use to construct the row?
CREATEREF
MULTISET
ROW
You have just set up the Entity Framework cascading delete, and you are now trying to use it, but you keep getting an UpdateException. What is the most logical cause of this exception?
You must make sure that all dependent objects are loaded
Your database does not support cascading deletes.
You have not defined a cascading delete at the database server.
You are working with an ObjectContext object that targets the mainframe and another ObjectContext object that targets SQL Server. When it’s time to save the changes, you want all changes to be sent to the mainframe and to SQL Server as one transaction. How can you accomplish this?
Just save both ObjectContext objects because they automatically join the same transaction.
Save to the mainframe and use an if statement to verify that the changes were successful. If successful, save to SQL Server.
Wrap the saving of both ObjectContext objects within a TransactionScope object that is implemented in a using statement in which the last line executes the Complete method on the TransactionScope class.
Use a Boolean flag to indicate the success of each save, which will tell you whether the save was successful
Which of the following keywords is used to implement eager loading of data when executing a query?
Expand
Value
Skip
Filter
You want to configure your WCF data service to allow only a single row lookup from the Customers entities and not allow queries that return all entities from Customers. How can you configure the WCF data service?
Config.SetEntitySetAccessRule(“Customers”, EntitySetRights.ReadSingle)
Config.SetEntitySetAccessRule(“Customers”, EntitySetRights.ReadMultiple)
Config.SetEntitySetAccessRule(“Customers”, EntitySetRights.AllRead)
The URI to your WCF data service is http://www.northwind.com/DataServices.svc. What can you add to the end of the URI to retrieve only a list of Order entities for the Customer entity whose CustomerID is ‘BONAP’?
Customers(‘BONAP’)?$expand=Orders
Customers?$filter=CustomerID eq ‘BONAP’&$expand=Orders
Customers/Orders?$filter=CustomerID eq ‘BONAP’
Customers(‘BONAP’)/Orders
You are writing a WCF data service that will be included in a large project that has many other WCF data services. Your WCF data service will provide access to a SQL server using the Entity Framework. The EDMX file already exists in the project and is used by other services. One of the tables exposed by your service is the Contacts table, which contains the list of employees and the list of external contacts, which are denoted by the IsEmployee column that can be set to 1 or 0. You want to configure your WCF data service to return the external contacts whenever someone queries the Contacts entity set through WCF Data Services. What is the best way to solve this problem?
Modify the Contacts entity set in the EDMX file.
Add a stored procedure to SQL Server and modify the EDMX file to create a function import that you can call.
Add a method to your WCF data service class and adorn the method with a QueryInterceptorAttribute of Contacts. In the method, provide the filter.
Add a lazy loader to the WCF data service that will filter out the employees.
Which payload formats can be specified if you are making an AJAX call to a WCF data service? (Each correct answer provides a complete solution. Choose two.)
SOAP
JSON
AtomPub
WSDL
When working with the Entity Framework, what can you use to retrieve the SQL of a query?
Add a TextWriter object to the Log property of ObjectContext
Set the Connection property of ObjectContext to a TextWriter object.
Use the ToTraceString method on the ObjectQuery object
. You create a PerformanceCounter object to view the SQL statements sent to the database server. True or false?
Ye
No
What must you use to capture an exception that might occur when you are sending changes to the database server?
A using block.
A try/catch block.
A while statement
You can’t capture exceptions.
Which of the following are valid encryption algorithms that can be selected when encrypting the connection strings in your .config files? (Each correct answer presents a complete solution. Choose two.)
DpapiProtectedConfigurationProvider
RNGCryptoServiceProvider
SHA256Managed
RsaProtectedConfigurationProvider
RijndaelManaged
Which of the following is a valid symmetric encryption algorithm?
RNGCryptoServiceProvider
RNGCryptoServiceProvider
SHA256Managed
RijndaelManaged
You want to synchronize data between your local SQL Server Compact tables and SQL Server 2008. You want the synchronization to send changes to SQL Server 2008, and you want to receive changes from SQL Server 2008. Which setting must you assign to the SyncDirection property of your sync agent’s tables?
SyncDirection.Bidirectional
SyncDirection.UploadOnly
SyncDirection.Snapshot
SyncDirection.DownloadOnly
You use Visual Studio .NET 2010 to create a WCF Data Services application that uses the ADO.NET Entity Framework to model entities. The WCF Data Services application uses the default model and mapping files that are deployed as application resources. After you deploy the application to the production server, you decide to update the conceptual model. What should you do?
You use Visual Studio .NET 2010 to create a WCF Data Services application that uses the ADO.NET Entity Framework to model entities. The WCF Data Services application uses the default model and mapping files that are deployed as application resources. After you deploy the application to the production server, you decide to update the conceptual model. What should you do?
Recompile the application and redeploy the modified assembly file
You use Visual Studio .NET 2010 to create a WCF Data Services application that uses the ADO.NET Entity Framework to model entities. You must ensure that the model and mapping files are not deployed as application resources. What should you do?
Modify the connection string in the application’s Web.config file to reference the absolute physical path to the EDMX file.
. Set the value of the EDMX file’s Metadata Artifact Processing property to Copy To Output Directory
Modify the connection string in the application’s Web.config file to reference the relative path to the model and mapping files.
{"name":"Baze", "url":"https://www.quiz-maker.com/QPREVIEW","txt":"Test your knowledge and skills in ADO.NET and LINQ with this comprehensive quiz! Whether you're a student, a developer, or just someone looking to learn more about data management in .NET, this quiz covers essential concepts and practical applications.You'll encounter a variety of topics, including:Data management techniquesWorking with SQL ServerLinq queries and methodsXML processing in .NET","img":"https:/images/course1.png"}