ES6, React and Redux
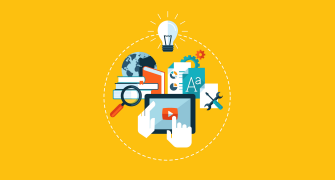
JavaScript and React Mastery Quiz
Test your knowledge on ES6, React, and Redux with this comprehensive quiz designed for developers of all skill levels. Whether you're a beginner or a seasoned pro, there's something here to challenge you.
Key Features:
- Multiple choice questions
- Real-world scenarios
- Instant feedback on your responses
Which keyword is NOT used to declare a variable in JS
Var
Let
Const
Val
Which of the following is NOT an arrow function?
X => x
Function(x){return x}
(x) => x
(x) => return x
Which code will return an array of [1,3,5]?
[1,2,3].map((x,i) => {alert(x); return x+i;})
[1,2,3].map((x,i) => {x+x; x+i;})
[1,2,3].map((x,i) => x*i)
[1,2,3].map((x,i) => {x*i+1;})
What is going to be the console output?
function f(x, y=x+3, z=2)
{
console.log(x+y+z);
}
{
console.log(x+y+z);
}
f(1);
f(1,null, 1);
f(1, undefined, 3);
f(1,null);
f(1,null, 1);
f(1, undefined, 3);
f(1,null);
7,6,8,7
6,6,8,6
7,2,8,3
7,6,4,7
7,2,4,3
const obj = {
prop1:1,
prop2:{
arr:[1,2,3]
},
arr:[1,2,3]
};
prop1:1,
prop2:{
arr:[1,2,3]
},
arr:[1,2,3]
};
We are going to use the object above for the following console output:
console.log(obj.prop1, obj.arr[1], obj.prop2.arr[0]);
You need to refactor(re-write) this line of code using ES6 object destructuring.
Which code from the options below is going to show an identical output(Choose as many answers as you want)?
Const {prop1, arr:[a,b], prop2:{arr:[c]}} = obj; console.log(prop1, b, c);
Const {prop1, arr:[a,b], prop2.arr:[c]} = obj; console.log(prop1, b, c);
Const {prop1, arr:[b], prop2:{arr:[c]}} = obj; console.log(prop1, b, c);
Const {prop1, {arr:[a,b]}, prop2:[arr:c]} = obj; console.log(prop1, b, c);
Const {prop1, arr:[a,...rest], prop2:{arr:[...p]}} = obj; console.log(prop1, rest[0], p[0]);
Const {prop1:p, arr:[a],arr:m,prop2:{arr:[c]}} = obj; console.log(p, m[0], c);
When you look at JSX code(the one inside return statement of the render function) what other language is it similar to?
Javascript
HTML
Java
French
It doesn't look like anything I ever seen
Which statement is correct?
Function component can have state unlike class component
Class component has an option to handle lifecycle events like componentDidMount unlike the function component
Function component can't have state and props
For class component, componentDidMount is called after the constructor but before render
The best place to change the state is in a render function of a class component
class Component1 extends Component
{
constructor()
{
super();
this.state= {
push:1,
pull:3
};
console.log("c");
}
componentDidMount()
{
console.log("m");
this.setState({push:2});
}
componentWillUnmount()
{
console.log("u");
}
render()
{
console.log("r");
return (
<div></div>
);
}
}
What is going to be the console output after Component1 is loaded on the page?
C,m,r,u
C,r,m,u
C,r,m
C,r,m,r
C,r,m,u,r
Component1 has the following code:
class Component1 extends Component
{
handlePullClick1()
{
console.log("handlePullClick1");
}
handlePullClick2()
{
console.log("handlePullClick2");
}
handlePullClick3 = (pull) => () =>
{
console.log("handlePullClick3");
}
render()
{
return (
<div>
<Component2 handlePullClick={this.handlepullclick1.bind(this)}handlePullClickArrow={this.handlepullclick3}>
{this.handlepullclick2.bind(this)}
</Component2>
</div>
);
}
}
Component2 has the following code:
class Component2 extends Component
{
onPullClick1()
{
//Line1
}
onPullClick2()
{
//Line2
}
onPullClick3()
{
//Line3
}
render()
{
return (
<div>
<Button onClick={() => this.onPullClick1()}>
Pull 1
</Button>
<Button onClick={this.onpullclick2.bind(this)}>
Pull 2
</Button>
<Button onClick={this.onpullclick3.bind(this)}>
Pull 3
</Button>
</div>
);
}
}
Replace commented lines with text Line1, Line2 and Line3 with the correct code that will call corresponding fun
This question should have 3 answers - 1 answer for each line
Line1:this.props.handlePullClick();
Line1: this.state.handlePullClick();
Line1: this.props.handlePullClick1();
Line2: this.props.handlePullClick2();
Line2: this.props.children();
Line2: this.props.children[0]();
Line3: this.props.handlePullClickArrow()();
Line3: this.props.handlePullClickArrow();
Line3: this.props.handlePullClick3();
<Switch>
<Route exact path="/">
<Route1 />
</Route>
<Route path="/:id">
<Route2 />
</Route>
<Route path="/route2">
<Route3 />
</Route>
<Route path="/route2/route3">
<Route4 />
</Route>
</Switch>
Given the routing configuration above, which component is going to be rendered when user navigates to /route2
Route1
Route2
Route3
Route4
Where do we store all the shared data(application state) in Redux?
Global JS object
Special Redux entity called "Store"
MVC model
Database
CSV file on disk
How would you best describe actions in Redux?
Functions of a store class
Events with payload of data to change the store
Functions that are directly changing the store
Classes that alerts DOM when something is changed
const obj = {
prop1:{
prop2:1
},
func: () => this.prop1.prop2 //Line #1
};
console.log(obj.func().bind(obj)); //Line #2
obj.func = function(){ //Line #3
return this.prop1.prop2; //Line #4
}
console.log(obj.func.bind(this)()); //Line #5
const obj1 = obj;
obj = obj1; //Line #6
Specify ALL lines that will cause an error(only those lines, that throw an exception to the console). Pseudo line numbers are specified in the comment. This is a multiple choice answer
Line #1
Line #2
Line #3
Line #4
Line #5
Line #6
Using component composition, we created several wrappers:
function ClassComposite(props)
{
return (
<div className="composite">
{props.children}
</div>
);
}
function PropertyComposite(props)
{
return (
<div className="property" {...props}>
{props.children}
</div>
);
}
function InjectPropertyComposition(BaseComponent)
{
return function() {
return(<BaseComponent prop1={3}/>);
}
}
Later on, in the render method of our main component, we have the following code:
const InjectedComposition = InjectPropertyComposition(PropertyComposite);
return (
<Fragment>
<ClassComposite prop2="2">
<InjectedComposition prop1={1}>
PropertyCompositeContent
</InjectedComposition>
<PropertyComposite prop1={1} prop2="2">
PropertyCompositeContent
</PropertyComposite>
</ClassComposite>
</Fragment>
);
What will be the HTML output of the main component?
<div class="composite">
<div class="property" prop1="3"></div>
<div class="property" prop1="1" prop2="2">PropertyCompositeContent</div>
</div>
<div class="composite">
<div class="property" prop1="1"></div>
<div class="property" prop1="1" prop2="2">PropertyCompositeContent</div>
</div>
<div class="composite">
<div class="property" prop1="3">PropertyCompositeContent</div>
<div class="property" prop1="1" prop2="2">PropertyCompositeContent</div>
</div>
<div class="composite">
<div class="property" prop1="1">PropertyCompositeContent</div>
<div class="property" prop1="1" prop2="2">PropertyCompositeContent</div>
</div>
<div class="property" prop1="1">PropertyCompositeContent</div>
<div class="property" prop1="1" prop2="2">PropertyCompositeContent</div>
</div>
<div class="composite">
</div>
</div>
<div class="composite">
<div class="property" prop1="3"></div>
<div class="property" prop1="1" prop2="2"></div>
</div>
<div class="property" prop1="3"></div>
<div class="property" prop1="1" prop2="2"></div>
</div>
In order to debug our Redux code, we put "console.log" statements in the key places of the application.
At the root of our app, in index.js we added the following code:
const store = createStore(rootReducer);
store.subscribe(() => console.log("Store updated"));
store.subscribe(() => console.log("Store updated"));
Index.js renders Component1 like that:
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root'));
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root'));
rootReducer,js has the following code:
const inititalState = {
push:1,
pull:2
}
export function rootReducer(state=inititalState, action)
{
switch(action.type)
{
case 'PUSH_DATA':
return {
...state,
push:action.push
};
case 'PULL_DATA':
return {
...state,
pull:action.pull
}
default:
return state;
}
}
push:1,
pull:2
}
export fun
{
switch(action.type)
{
case 'PUSH_DATA':
return {
...state,
push:action.push
};
case 'PULL_DATA':
return {
...state,
pull:action.pull
}
default:
return state;
}
}
Finally, Component1 has the following code:
class Component1 extends Component
{
componentDidMount()
{
this.props.onPull(3);
}
render()
{
return (<div>{this.props.push}</div>);
}
}
{
componentDidMount()
{
this.props.onPull(3);
}
render()
{
return (<div>{this.props.push}</div>);
}
}
//Actions
function pushData(push)
{
console.log("pushData Action");
return {
type: "PUSH_DATA",
push: push
};
}
{
console.log("pushData Action");
return {
type: "PUSH_DATA",
push: push
};
}
export function pullData(pull)
{
console.log("pullData Action");
return {
type: "PULL_DATA",
pull: pull
}
}
{
console.log("pullData Action");
return {
type: "PULL_DATA",
pull: pull
}
}
const mapStateToProps = state => {
console.log("mapStateToProps", state.push, state.pull);
return {
...state
}
};
console.log("mapStateToProps", state.push, state.pull);
return {
...state
}
};
const mapDispatchToProps = dispatch => {
console.log("mapDispatchToProps");
return {
onPull: (payload) => {
console.log("onPull");
return dispatch(pullData(payload));
}
};
};
console.log("mapDispatchToProps");
return {
onPull: (payload) => {
console.log("onPull");
return dispatch(pullData(payload));
}
};
};
export default connect(mapStateToProps, mapDispatchToProps)(Component1);
What text is going to be in the console once Component1 is rendered?
mapStateToProps 1 2;mapDispatchToProps; onPull; pullData Action; Store updated; mapStateToProps 1 3
mapStateToProps 1 2; onPull; mapDispatchToProps; pullData Action; Store updated; mapStateToProps 1 3
mapStateToProps 1 2; onPull; mapDispatchToProps; pullData Action; Store updated;
mapStateToProps 1 2; mapDispatchToProps; onPull; pullData Action; Store updated;
{"name":"ES6, React and Redux", "url":"https://www.quiz-maker.com/QPREVIEW","txt":"Test your knowledge on ES6, React, and Redux with this comprehensive quiz designed for developers of all skill levels. Whether you're a beginner or a seasoned pro, there's something here to challenge you.Key Features:Multiple choice questionsReal-world scenariosInstant feedback on your responses","img":"https:/images/course4.png"}