Set 2-1
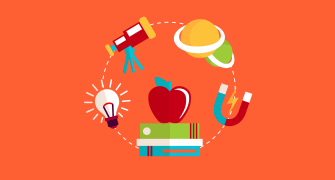
Web Development Quiz: Test Your Skills!
Challenge yourself with our comprehensive web development quiz designed to test your knowledge across various topics including JavaScript, APIs, and Node.js.
Features:
- 10 Challenging Questions
- Multiple Choice and Checkbox Formats
- Instant Feedback on Your Answers
Refer to the following code:
01 class Ship {
02 constructor(size) {
03 this.size = size;
04 }
05 }
06
07 class FishingBoat extends Ship {
08 constructor(size, capacity) {
09 // Missing code
10 this.capacity = capacity;
11 }
12 displayCapacity() {
13 console.log('The boat has a capacity of $(this.capacity) people.');
14 }
15 }
16
17 let myBoat = new FishingBoad('medium', 10);
18 myBoat.displayCapacity();
Which statement should be added to line 09 for the code to display 'The boat has a capacity of 10 people'?
Super.size = size;
Ship.size = size;
Super(size);
This.size = size;
Which 3 browser specific APIs are available for developers to persist data between page loads?
Global variables
IIFEs
LocalStorage
Cookies
IndexedDB
In the browser, the document object is often used to assign variables that require the broadest scope in an application. Node.js application do not have access to the document object by default.
Which two methods are used to address this?
Choose 2 answers
Assign variables to module.exports and require them as needed
Use the window object instead
Create a new document object in the root file
Assign variables to the global object
A developer is wondering whether to use, Promise.then or Promise.catch, especially when a promise throws an error.
Which two promises are rejected?
Choose 2 answers
Promise.reject('Cool error here').catch(error => console.error(error));
Promise.reject('Cool error here').then(error => console.error(error));
New Promise(() => (throw 'Cool error here')).then((null, error => console.error(error)));
New Promise((resolve, reject) => (throw 'Cool error here')).catch(error => console.error(error));
A developer wants to set up a web server that handles HTTP/2 with Node.js. The developer created a directory locally called app-server, and the first file added is index.js (at app-server/index.js).
Without using any third-party libraries, what should the developer add to line 1 of index.js?
Const http2 = require('http2');
Const server = require('http-2');
Const tls = require('tls');
Const https = require('https');
class Student {
constructor(name) {
this.name = name;
}
takeTest() {
console.log(`$(this.name) got 70% on test.');
}
}
class BetterStudent extends Student {
constructor(name) {
super(name);
this.name = 'Better student ' + name;
}
takeTest() {
console.log(`$(this.name) got 100% on test.');
}
}
let student = new BetterStudent('Jackie');
student.takeTest();
What is the console output?
> Uncaught ReferenceError
> Better student Jackie got 70% on test.
> Better student Jackie got 100% on test.
> Jackie got 70% on test.
Refer to the following array:
let arr = [1, 2, 3, 4, 5];
Which three options result in x evaluating as [1, 2]?
Let x = arr.slice(2);
Let x = arr.slice(0, 2);
Let x = arr.filter((a) => { return a<= 2 });
Let x = arr.filter((a) => { a<= 2 });
Let x = arr.splice(0, 2);
Refer to the following code:
01 let obj = {
02 foo: 1;
03 bar: 2;
04 }
05 let output = [];
06
07 for (let something of obj) {
08 output.push(something);
09 }
10
11 console.log(output);
What is the value of output on line 11?
[1, 2]
["foo", "bar"]
["foo:1", "bar:2"]
An error will occur due to the incorrect usage of the for..of statement on line 07.
A developer has two ways to write a function:
Option A:
function Monster() {
this.growl = () => {
console.log("Grr!");
}
}
Option B:
function Monster() {};
Monster.prototype.growl = () => {
console.log("Grr!");
}
After deciding on an option, the developer creates 1000 monster objects.
How many growl methods are created with Option A and Option B?
1000 growl methods are created for Option A. 1 growl method is created for Option B.
1000 growl methods are created regardless of which option is used.
1 growl method is created for Option A. 1000 growl methods are created for Option B.
1 growl method is created regardless of which option is used.
{"name":"Set 2-1", "url":"https://www.quiz-maker.com/QPREVIEW","txt":"Challenge yourself with our comprehensive web development quiz designed to test your knowledge across various topics including JavaScript, APIs, and Node.js.Features:10 Challenging QuestionsMultiple Choice and Checkbox FormatsInstant Feedback on Your Answers","img":"https:/images/course5.png"}