Inheritance
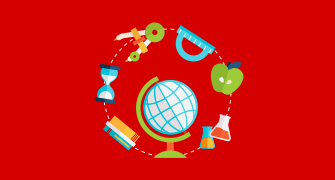
Understanding Inheritance in Object-Oriented Programming
This quiz is designed to test your knowledge of inheritance, one of the fundamental concepts of object-oriented programming. Whether you're a student or a professional brushing up on your skills, this quiz will challenge you.
You'll encounter questions covering:
- Superclasses and subclasses
- Accessors and mutators
- Encapsulation concepts
- Real-world application scenarios
- Code behavior and expected outputs
What is a mutator?
public class A {
public int al;
public void methodA() {
bl = 0; // Statement I
}
public void methodC() {
al = 0;
}
}
public class B extends A {
public int bl;
public void methodB() {
methodC(); // Statement II
al = 0; // Statement III
}
}
Which of the labeled statements in the methods shown above will cause a compile-time error?
A: public class Drink extends Refrigerator {
private Soda mySoda;
…
}
…
}
C: public class Drink extends Soda {public class Drink extends Soda
}
public class Refrigerator {
private Drink[] myDrinks;
…
}
D: public class Soda extends Drink {…
}
public class Refrigerator {
private Drink[] myDrinks;
…
}
E: public class Drink extends Drink {public Drink drink;
}
public class Super {
public String toString() {
return "super";
}
}
public class Duper extends Super {
public String toString() {
return “duper";
}
}
public class SuperDuper extends Duper {
public String toString() {
return super.toString() + "duper";
}
}
What is output to the console when the following code is executed?
SuperDuper sd = new SuperDuper();
System.out.println(sd.toString());
public class Student {
public String getFood() {
return "Pizza";
}
public String getInfo() {
return this.getFood();
}
}
public class GradStudent extends Student {
public String getFood() {
return "Taco";
}
}