IAPP001 Multiple Choice
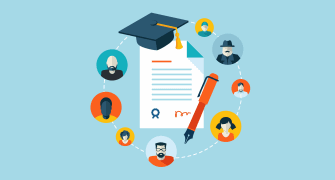
Mastering Java Concepts: A Comprehensive Quiz
Test your knowledge of Java programming concepts with this engaging quiz designed for enthusiasts and professionals alike. Covering topics such as OOP principles, design patterns, and method overloading, this quiz will challenge your understanding and reinforce your learning.
- 78 thought-provoking questions
- Multiple choice, text, and checkbox formats
- Assess your skills and knowledge
Using the following code: 1 public abstract class ClassA 2 { 3 protected int variable1; 4 public ClassA(int a) 5 { 6 variable1 = a; 7 } 8 public abstract void method1(); 9 public String method2() 10 { 11 return "Something"; 12 } 13 }
1 public class ClassB extends ClassA 2 { 3 public ClassB(int number) 4 { 5 super(number); 6 } 7 public void method1() 8 { 9 ++variable1; 10 } 11 public int method1(int y) 12 { 13 return (int)Math.pow(y, 3); 14 } 15 public String method2() 16 { 17 return "Something else"; 18 } 19 }
What type of inheritance is used? |
Using the following code:
- public abstract class ClassA
- {
- protected int variable1;
- public ClassA(int a)
- {
- variable1 = a;
- }
- public abstract void method1();
- public String method2()
- {
- return "Something";
- }
- }
- public class ClassB extends ClassA
- {
- public ClassB(int number)
- {
- super(number);
- }
- public void method1()
- {
- ++variable1;
- }
- public int method1(int y)
- {
- return (int)Math.pow(y, 3);
- }
- public String method2()
- {
- return "Something else";
- }
- }
What type of inheritance is used?
Given the following method signatures:
setLength(double length)
setLength(String length)
This is an example of overloading
Given the following method signatures: setLength(double length) setLength(String length)
This is an example of overloading |
In the following code example, which is the only polymorphic line of code? public class Shapes public Shapes() |
Consider the following code:
Animal animal;
animal.getName();
What is wrong this this code?