So1
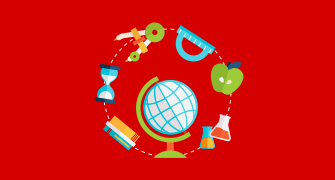
Memory Management Mastery Quiz
Test your knowledge on memory management, memory segments, and page faults with this comprehensive quiz designed for programmers and computer science enthusiasts. Challenge yourself with 59 carefully crafted questions that dive deep into the intricacies of computer memory.
- Questions cover a range of topics including memory allocation and segmentation faults.
- Perfect for students, teachers, and anyone looking to refresh their understanding of computer memory.
How can we allocate memory in the `.stack` section?
Declare a variable in a function.
Use the `malloc` function family.
Use the `static` keyword.
The stack is only modified by the OS, so we can't influence it.
How do cache misses influence our program?
They add latency to read/write operations.
They corrupt our memory.
They lead to reading the wrong data.
They lead to writing the wrong data.
Can a process access the memory of another process?
Yes, by using shared memory zones.
Yes, by directly accessing the memory.
Yes, by making changes in the physical memory.
No, there is no such mechanism.
Which of the following is a *disadvantage* of using memory pages?
It may cause memory fragmentation.
It makes accessing memory easier.
It makes accessing memory harder.
It makes addressing memory harder.
What is the most likely reason our process didn't stop, even though a page fault occurred?
A minor page fault occurred; the memory page was mapped in the address space of the process.
A major page fault occurred; we ignored it.
A medium page fault occurred; it requires no intervention.
No page fault actually occurred.
Which of the following triggers a major page fault?
The page we want to access was swapped out.
The page we want to access is allocated in RAM.
An `mprotect()` call was made.
A `free()` call was made.
Which of the following triggers a page fault, but not a segmentation fault?
`malloc(100GB)`.
Writing in `.rodata`.
Executing code from stack.
Reading from an unmapped page.
What is the role of the TLB?
It plays a role similar to the CPU cache.
It guarantees the existence of pages in memory.
It is part of the CPU architecture.
It is part of the HDD.
What is the role of the MMU?
Checks whether virtual addresses are valid.
Handles reading/writing to disk.
Guarantees coherent data is written to disk.
It is a software component of the OS.
What happens after a page fault is resolved successfully?
The last instruction is executed again.
The program quits its execution.
The program is restarted from the beginning.
The last instruction is skipped and execution continues normally.
Which of the following is *NOT* an operation performed by the page fault handler?
Handles the TLB Cache.
Verifies if an address is valid.
Issues segmentation faults.
Updates the page table with new entries.
What is the main purpose of swapping?
It evicts frames to/from the disk to increase/decrease the RAM.
It swaps virtual addresses for physical ones.
It swaps physical addresses for virtual ones.
It enables hot-swapping of applications.
What is a tuple we use to describe memory zones?
Address, Size, Access rights
OS, MMU, TLB
Register, Cache, RAM
Virtual Address, Cache Address, Physical Address
What can this `const char *` be translated into?
A pointer to a string of constant elements
A constant pointer to a string
A string
A constant pointer to a string of constant elements
How can we allocate memory in the `.heap` section in Python?
We do not handle memory zones in Python.
We use the `malloc` family equivalent in Python.
We use the `-h` flag when starting Python.
We use the `h` flag when allocating memory.
What happens when we access a memory zone for which we have no rights?
A `Segmentation Fault` error is received.
A `Page Fault` warning is received.
An `Access Restricted` error is received.
The operation fails silently.
What happens when we read memory from an expired stack frame in C?
The access is valid, but the data inside might or might not be altered in the meantime.
The access is valid and we retrieve our data.
The access results in a `Segmentation Fault` error.
The access results in a `Stack Smashing` error.
How many `Segmentation Fault` errors are generated when accessing element 100 in a 10-element list in Python?
0
1
90
99
What is the use of the `pmap` tool?
It is used to see the memory layout of a process.
It is used to map physical memory.
It is used to map process memory.
It is used to see the memory layout of a file.
Why can we sometimes run code saved in the `.rodata` region?
The zone is coalesced in the `.text` region.
The zone is read-only.
The zone can be forced to have execution rights when the process starts.
The `.rodata` zone was randomly given execution permissions at runtime.
Which loads faster between a dynamic and a static executable?
A dynamic executable
A static executable
They load in the same time frame.
Loading does not depend on the executable type.
How many stacks does an 8-threaded program have?
8; one for each thread.
1; one for all threads.
4; one for every 2 threads.
2; one for the main thread, one for the others.
How many `malloc` system calls are made after calling it 10 times in a loop?
`malloc` is not a system call.
1, as they are merged together.
10, once for each `malloc` call.
Somewhere between 1 and 10.
What is the main difference between `brk` and `mmap`?
`mmap` is used for larger memory allocations.
`brk` also inserts a 'page break' in the page table.
`brk` is used for larger memory allocations.
`mmap` is used only on minor page faults.
What is the main difference between reading using `mmap` and `read`?
`mmap` lets the system handle the reading from file.
`read` called repeatedly is faster.
`mmap` uses the `read` syscall underneath.
`mmap` is easier to use.
What does 'Resident Set Size' refer to?
The size of the physical memory used.
The size of the virtual memory used.
The size of the set of instructions residing in alternate memory states.
The size of the set of indirect pointers referring to alternate memory states.
Can we write to a `const` variable that was allocated on the *stack*?
Yes, but we need to cast the variable first.
Yes, we just assign a new value (e.g., `x = 3`).
No, constant variables can never be modified.
No, because the page permission is read-only.
How do we deallocate a variable in the .stack section?
We can't, as we need to wait to go out of scope.
We can't, as they are constant and kept in memory forever.
We can, as the stack is writable.
We can, as the .stack section can be resized with `brk`/`sbrk` calls.
What is a characteristic of the 'still reachable' section in Valgrind?
It refers to allocated memory that is still pointed to when the process finished.
It refers to allocated memory that is no longer pointed to.
It refers to the stack memory, which can't be freed with calls to `free`.
It refers to memory lost after using the `brk`/`sbrk` calls directly.
What happens with memory leaks after a process finishes?
The whole virtual memory is cleared after finishing, along with memory leaks.
The memory leaks remain until a reboot.
The memory leaks stay forever.
After finishing, the OS clears only the memory that it can reach, so the memory is lost.
When are system calls required?
When performing privileged operations.
When calling library functions.
When copying strings.
When the system notifies the process that an event has happened.
What instruction, used for performing system calls, is available only in the assembly language (i.e., is not inserted by a compiler for regular functions) that makes using wrapper functions like `read` and `write` mandatory?
Syscall
Leave
Ret
Rot
How does the system identify which system call is performed?
A numeric value, written in `rax`.
A pointer to a string, written in `rdx`.
The first four letters of the system call name, written in `rbx`.
The name of the wrapper function used to perform the system call, written in `rax`.
What system call is ultimately used by `printf` to print data to the standard output?
Write
Read
Mmap
Open
What system call is ultimately used by `scanf` to get data from the standard input?
Read
Write
Mmap
Open
How many system calls must be performed by the following code? ``` void copy(void *dest, const void *src, int len) { int I; for (i = 0; I < len; i++) memcpy(dest, src, 1); } ```
0
Len
Len / 2
1
How many system calls are performed by the following code? ``` for (i = 0; I < N; i++) write(1, "ab", 2); ```
N
0
2 * N
1
The operating system's kernel does NOT:
Update system packages.
Manage access to hardware resources.
Isolate processes.
Provide a hardware abstraction layer.
What information about a process can we obtain using `strace`?
What system calls it performs.
What library calls it performs.
How many functions it calls.
If it contains memory leaks.
What information about a process can we obtain using `ltrace`?
What library calls it performs.
What system calls it performs.
How many functions it calls.
If it contains memory leaks.
What is an advantage of using dynamic libraries as opposed to static libraries?
The resulted binary file is smaller.
The code requires fewer function calls.
It is easier to run the application on other systems.
The code is easier to read.
What is an advantage of using dynamic libraries as opposed to static libraries?
The library's code is loaded only once in memory.
The code requires fewer function calls.
Portability.
The code is easier to read.
Why will `printf("Hello")` usually not perform a system call?
The string is buffered in `stdout`'s buffer.
`printf` does not need a system call to output data.
System calls are only required if more than 32 bytes of data is printed.
The standard output was redirected to `/dev/null`.
Why does `printf` buffer the strings instead of immediately displaying them (unless a new line is also printed)?
To minimise the overhead caused by calling `write` multiple times.
To minimise the overhead caused by calling `read` multiple times.
To log the output messages in an internal log.
To reduce the number of times the standard output file is open.
How many times is a dynamic library's code loaded in the system's physical memory?
1.
0.
Once for each application that uses that library.
Once for each thread that uses that library.
How many times is a static library's code loaded into the system's memory?
1.
0.
Once for each application that uses that library.
Once for each thread that uses that library.
Which of the following is a similarity between a C application and a C library?
Both are binary files.
Both have an entry point.
Both use libc.
Both are portable.
Which of the following is a difference between an application and a library?
The application has an entry point, while the library does not.
The application is a binary file, while the library is a text file.
The library has an entry point, while the application does not.
The application is reusable, while the library is not.
Which of the following is a functionality of an operating system?
Isolation between processes.
Code compilation.
String operations.
Software package installation.
{"name":"So1", "url":"https://www.quiz-maker.com/QPREVIEW","txt":"Test your knowledge on memory management, memory segments, and page faults with this comprehensive quiz designed for programmers and computer science enthusiasts. Challenge yourself with 59 carefully crafted questions that dive deep into the intricacies of computer memory.Questions cover a range of topics including memory allocation and segmentation faults.Perfect for students, teachers, and anyone looking to refresh their understanding of computer memory.","img":"https:/images/course8.png"}
More Quizzes
OS Practice Day 1
24120
Sheet-7 (OS)
3015233
Quiz # 01 _ Operating-System-Concepts _ By Engr. Ahmad Saeed
12612
CS 446 Practice
512649
Mapping Functions Quiz
15811
Operating Systems Quiz CTS Students CLub
16825
CS 332 Practice Exam
361821
Dsa
15825
Quiz # 01 _ DS&A _ Spring 2020 _ By Engr. Ahmad
12612
Operating System
1050
Os chapter 3
1058
CECS 326 Final Part 3
25129