Software Techniques
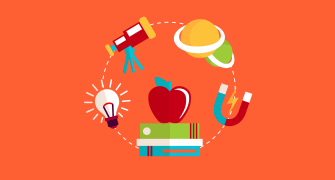
Mastering C# and Windows Forms Quiz
Test your knowledge of C# and Windows Forms with our comprehensive quiz designed for developers of all levels. Challenge yourself with questions that cover essential concepts in software development, threading, and UI design.
Get ready to explore:
- C# Basics
- Windows Forms Architecture
- Threading and Synchronization
- Event Handling
When building C# code, the first step is to compile it to an intermediate language representation (MSIL). This requires further compilation before it can be executed.
True
False
In Windows Forms, Controls can be organized into a parent/child, as well as an Owner/Owned relationship.
True
False
N Windows Forms, Forms can be organized into a parent/child, as well as an Owner/Owned relationship.
True
False
In Windows Forms, Controls can be organized into parent/child.
True
False
In Windows Forms, Controls can be organized into parent/child, while Forms can be organized into owner/owned relationships.
True
False
We want a Windows Forms Form to redraw itself. Instead of calling OnPaint, we must call … method so that the redrawing is scheduled by the OS?
In C#, interfaces may contain methods, fields (member variables) and properties
True
False
You can subscribe to a C# event using the = operator
True
False
You can subscribe to a C# event using the += operator
True
False
A thread can wait for another thread to finish by calling its Join() method
True
False
In C#, you must register at least one event handler for each event.
True
False
In C#, delegate objects are the modern equivalent to C function pointers. However, unlike in C, they can reference/point to multiple functions.
True
False
What class should we use in .NET, if we have a thread that wants to signal several other threads that it has finished reading data from a file. All of the waiting threads should respond to the signal.
In a Windows Forms application, we want to create a complex (made up of multiple controls) reusable control. What is the best way to achieve this?
In .NET, the Semaphore has the advantage compared to the Mutex that it can be used to synchronize threads of different processes, whereas the Mutex can only be used inside a single process.
True
False
In Win32 applications, messages always go into the target window’s message queue before being handled.
True
False
In Java, we use the “@AttributeName”syntax, in C# the “[AttributeName]” syntax to declaratively add annotations to classes.
True
False
In Win32 applications, every window has a message loop that retrieves and dispatches messages from its queue.
True
False
The JIT compiler compiles intermediate language code to native machine code.
True
False
We can rotate drawings (e.g. text) in 2D space in Windows Forms applications.
True
False
Windows Forms supports rotating figures (e.g. Bitmaps or text) in 3 dimensions (3D).
True
False
We are writing a .NET server application that must serve a large number of clients at any given time. Provide the .NET class/concept that is most suitable for providing efficient parallel access to the clients. 1-2 words
A process will exit when there are no more running foreground threads. Background threads are ignored from this perspective.
True
False
A process will exit when there are no more running background threads. Foreground threads are ignored from this perspective.
True
False
Whenever an invalid area is created in our Windows Forms application (for example by another window being dragged off of it) ,we must call invalidate to redraw the area
True
False
Just like Java .class files, each .NET assemblies contains the IL code representation of a single class
True
False
Unlike Java .class files, each .NET assemblies may contain the IL code representation of multiple classes.
True
False
Applications compiled for the full .NET Framework cannot be run on Linux without using Wine or other emulation tools.
True
False
We place a Panel on a Windows Forms Form in such a way that the Panel and the Form’s edges have some space between them. What is the easiest way to achieve the effect, where if the Form’s height is changed, the Panel’s height also changes by the same amount?
Docking
Grid Panel
Anchoring
Splitter
Sticky Edge
When we set the DialogResult property of a dialog window, it is closed automatically.
True
False
.NET supports multiple programming languages
True
False
When building c# code, the first step is to compile it to native machine languages instructions.
True
False
In .Net we use a lock instruction with the same object parameter inside both functions foo() and bar(). We also know that foo() calls bar(). In other words, we have a case of recursive locking. Evaluate the statement: when foo() is called from any thread, it will cause a deadlock.
True
False
N .Net, we want to retrieve from a worker thread the content of the textbox’s text property (this was instantiated in the main thread). What mechanism/method must we use?
In win32, messages go to system-level queue, before being dispatched to an application queue
True
False
The basic scenario we refer to as DLL Hell : a new application is installed, which overwrites a DLL used by another application. This can happen because the file name of the two DLLs is the same.
True
False
In .NET, the semaphore has the advantage compared to the Mutex that it can be used synchronize threads of different processes, whereas the Mutex cannot.
True
False
What class should we use in .Net, if we have a thread that wants to signal another thread that it has finished reading data from a file. Only one thread should respond to the signal.
In win32 applications we use a switch-case structure in the message loop to react to events.
True
False
-- In a .NET application we ‘circumvent’ .NET and write a class that uses the native Win32 API to reserve resources (e.g. Open files). We write a dispose method for this class, since this allows the resources to be freed as soon as they are not needed
True
False
The JIT compiler takes C# code and compiles it to the native machine language instructions.
True
False
In c# we use the delegate keyword to define an event inside a class.
True
False
The win32 message created for a keyboard button press contains the code of the button that was pressed in the HWND hwnd field.
True
False
In a ‘connectionless’ data access model, we do not create a database connection when saving data back to the database.
True
False
In a connectionless data access model, we only connect to the database once, when we save the user’s changes
True
False
In a connectionless data access model, we have to keep a record of user’s changes in memory, despite the model’s central idea being that changes are saved to the database as soon as they are made
True
False
NET assemblies (exe , dll) contain machine language instructions.
True
False
In c# when we call a delegate object, all functions registered to the delegate will be called in order
True
False
The .Net runtime environment is abbreviated as CLS ( Common Language Specification) .
True
False
We can rotate drawings (e.g text) in 2D space in windows form application.
True
False
In Win32 message created for a keyboard button press contains the code of the button that was pressed.
True
False
The processing of Windows events for an application happens in parallel inside an application
True
False
In c# the delegate keyword defines a new type, from which can initiate a local variable.
True
False
In windows form modal dialog window can signal whether the user closed them using OK or Cancel buttons by setting DialogResult.
True
False
Private .Net assemblies are more secure, because they are signed with the publisher’s secret key
True
False
A win32 CALLBACK function is a special function type implemented by the OS that we call from our application
True
False
In a Win32 environment, every window has a CALLBACK function to handle the window’s messages.
True
False
Application developed using .NET core can also run on Linux.
True
False
In Win32 application, we typically use a switch-case structure in the window procedure to react to events.
True
False
In .NET, incrementing a 32 bit integer using operator++ is thread-safe.
True
False
In .NET, incrementing an 8 bit integer using operator++ is not thread-safe.
True
False
Most modern operating systems use non preemptive thread scheduling to avoid starving threads of CPU time.
True
False
In a C# class, we store a large Array or List of strings. We must write a destructor and a Dispose function to make sure that our Array or List is freed.
True
False
In a C# class, we store a large Array initializing it in the constructor. We must write a destructor and a Dispose function to make sure that the Array is freed.
True
False
When building C# code, the first step is to compile it to MSIL. This requires further compilation before it can be executed
True
False
If a class is thread-safe, it can be used from multiple threads without any further synchronization.
True
False
In .NET, a process waits for all of its foreground and background threads to finish running before it exits.
True
False
Win32 API functions can be called from multiple programming environments, including those provided by .NET
True
False
In .NET, we need a locking solution that allows N parallel accesses and works between processes. What mechanism/class would you use?
The language of choice for developing using the Win32 API is C#.
True
False
When using the C# lock statement, we must place it inside a using block (or alternatively, call CloseI() explicitly in a finalize block)
True
False
In Windows Forms, to separate code that was hand-written from auto-generated code, we use ……. Classes.
Installing private .NET assemblies is simple. You just have to copy them onto the machine (i.e you don’t need to register them with the OS)
True
False
We are writing a multi-threaded .NET application in which one thread tries to acquire locks first on object X, then on object Y. A second thread tries to acquire locks first on object Y, then object X. What kind of danger does this pose (one word)?
From the three studied techniques to create custom controls, all the three create controls which can be drag-and-dropped from the visual studio Toolbox
True
False
From the three studied techniques to create custom controls, only solutions that involve classes derived from UserControl class can be drag-and-dropped from the visual studio Toolbox. F
True
False
. In .NET if the internals of a class defined in a DLL change (e.g. We add a private member variable) while its interface stays the same, then we must recompile both the DLL and the components that use the DLL.
True
False
{"name":"Software Techniques", "url":"https://www.quiz-maker.com/QPREVIEW","txt":"Test your knowledge of C# and Windows Forms with our comprehensive quiz designed for developers of all levels. Challenge yourself with questions that cover essential concepts in software development, threading, and UI design.Get ready to explore:C# BasicsWindows Forms ArchitectureThreading and SynchronizationEvent Handling","img":"https:/images/course5.png"}