.NET Test
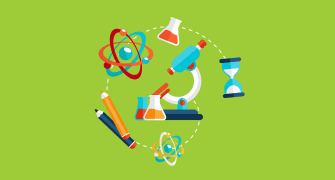
DotNET Mastery Quiz
Test your knowledge of .NET programming with our engaging quiz! Dive into questions covering various aspects of .NET, from multithreading to best practices in coding. Whether you're a beginner looking to learn or an expert wanting to brush up on your skills, this quiz is designed for you.
- Multiple-choice questions to assess your understanding.
- In-depth topics highlighting core .NET concepts.
- Immediate feedback on your performance!
What is the difference between the ref and out keywords?
Variables passed to out specify that the parameter is an output parameter, while ref specifies that a variable may be passed to a function without being initialized.
Variables passed to ref can be passed to a function without being initialized, while out specifies that the value is a reference value that can be changed inside the calling method.
Variables passed to out can be passed to a function without being initialized, while ref specifies that the value is a reference value that can be changed inside the calling method.
Variables passed to ref specify that the parameter is an output parameter, while out specifies that a variable may be passed to a function without being initialized.
What is this code an example of?
private static object objOne;
private static object objTwo;
private static void performTaskOne()
{
lock (objTwo)
{
Thread.Sleep(1000);
lock (objOne) {
} }
}
private static void PerformTaskTwo()
{
lock (objOne)
{
lock (objTwo) {
} }
}
A private class that uses multithreading
Multithread coding
Thread mismanagement
A potential deadlock
Which of its members must be implemented, comply with rules for the following interface? ?
public interface IAddress
{
string FirstLine { get; set; }
string LastLine { get; }
}
Both the FirstLine and LastLine properties need to be implemented.
Neither, they are both optional.
Only the LastLine property needs to be implemented.
Only the FirstLine property needs to be implemented.
During code execution what will the console ouput?
public delegate void SSOCallback(bool validUser);
public static SSOCallback ssologinCallback = SSOLogin;
public static void SSOLogin()
{
Console.WriteLine("Valid user!");
}
public static void Main(string[] args)
{
ssologinCallback(true);
}
Login successful...
Valid user!
An error, because the method signature of SSOLogin doesn't match the delegate
Login successful... Valid user!
What is the output of the program below?
public class TestStatic {
public static int TestValue;
public static int TestValue;
public TestStatic() {
if (TestValue == 0) {
TestValue = 5;
}
}
static TestStatic() {
if (TestValue == 0) {
TestValue = 10;
}
if (TestValue == 0) {
TestValue = 5;
}
}
static TestStatic() {
if (TestValue == 0) {
TestValue = 10;
}
}
public void Print() {
if (TestValue == 5) {
TestValue = 6;
}
Console.WriteLine("TestValue : " + TestValue);
if (TestValue == 5) {
TestValue = 6;
}
Console.WriteLine("TestValue : " + TestValue);
}
}
}
public void Main(string[] args) {
TestStatic t = new TestStatic();
t.Print();
}
t.Print();
}
TestValue : 6
TestValue : 10
TestValue : 5
TestValue : 0
What is .NET?
.NET is a general-purpose programming language. The language has expanded significantly over time, and now has object-oriented, generic, and functional features in addition to facilities for low-level memory manipulation.
.NET is a virtual machine that enables a computer to run programs written in several languages and compile programs to bytecode.
.NET is an interpreted, high-level, general-purpose programming language. Its language constructs an object-oriented approach aimed at helping programmers write clear, logical code for small and large-scale projects.
.NET is a free, cross-platform, open-source developer platform for building many different types of applications with multiple languages, editors, and libraries for web, mobile, desktop, gaming, and IoT.
What does the code shown below demonstrate, and why?
static void Multiply(int num1, int num2) {};
static void Multiply(double num1, double num2, double num3) {};
static void Multiply(float num1, float num2) {};
Polymorphism, because each method can perform different task
Method overriding, because it display the same method name, different or same parameters, and same return type
Method overloading, because it allows the creation of several methods with the same name, wich differ by the type of input via parameter
Method overriding, because it display the same method name, different parameters, and same return type
Which of the following is true about multitasking thread?
Thread multitasking allows code to be executed concurrently
Thread multitasking allows code to be executed only when handling a user event.
Thread multitasking blocks code from being executed simultaneously to guard memory.
Thread multitasking adds single-threaded code blocks together.
Find out major difference between finalize and finally blocks?
The finally block is called during the execution of a try and catch block, while the finalize method is called after garbage collection.
The finally block is called after the execution of a try and catch block, while the finalize method is called just before garbage collection.
The finalize block is called before the execution of a try and catch block, while the finally method is called just before garbage collection.
The finalize block is called during the execution of a try and catch block, while the finally method is called after garbage collection.
What is the output of the program below?
delegate void Scanner();
static void Main()
{
List<Scanner> scanners = new List<Scanner>();
int i=0;
for(; I < 10; i++)
{
scanners.Add(delegate { Console.WriteLine(i); });
}
foreach (var scanner in scanners)
{
scanner();
}
}
The number 10 ten times
The number 1 to 10
0
An error, because the method signature of SSOLogin doesn't match the delegate
{"name":".NET Test", "url":"https://www.quiz-maker.com/QPREVIEW","txt":"Test your knowledge of .NET programming with our engaging quiz! Dive into questions covering various aspects of .NET, from multithreading to best practices in coding. Whether you're a beginner looking to learn or an expert wanting to brush up on your skills, this quiz is designed for you.Multiple-choice questions to assess your understanding.In-depth topics highlighting core .NET concepts.Immediate feedback on your performance!","img":"https:/images/course6.png"}