Knowledge Check 5 Reviewer
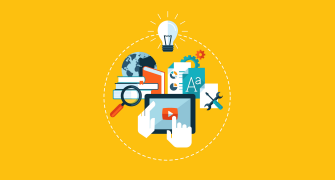
Ultimate Strings and Templates Knowledge Check
Test your understanding of strings, templates, and the C++ programming language with our comprehensive quiz. Dive deep into the fundamental concepts and see how well you can apply your knowledge in a challenging 60-question format!
- Explore key fun
ctions and members associated with strings. - Understand the intricacies of templates and their applications.
- Perfect for learners and developers seeking to enhance their skills.
Consists of arrays of characters and methods useful for managing them.
Arrays
Vectors
Strings
Pointers
Strings offer unbounded length because the memory allocation is done at runtime.
True
False
The length of the string is found using member function:
Strlen()
length()
String()
Len()
Individual characters begin with zero like arrays, so to access the last character in a string, the reference number would be the length minus 1.
True
False
Strings function similarly to pointers and are thus the same.
True
False
What are the benefits of using strings?
String objects manage the memory allocation and storage automatically.
String objects offer unlimited length because the memory allocation is done at runtime.
String objects consist of many member functions.
All of the above.
None of the above.
In the initialization /* string s2( 50, 'x' ); */ , what is the code processing?
S2 allows ‘x’ character to be stored 50 times
S2 allows ‘x’ character to be printed 50 times
S2 runs the calculation of ‘x’ characters 50 times
S2 allows the value '50' to be stored in x
Getline (streamName,Str) is a function used to read text into a string.
True
False
This member function allows you to search for a character or group of characters within a given string.
Search
Locate
Find
This
The function length of the string class returns the result in what type?
Void
Character
Unsigned integer
String
A series of bytes is known as?
Megabytes
Gigabytes
String
Stream
Which of the following is NOT a benefit of stream usage?
Streams provide a flexible, portable, and reusable set of tools for development of UNIX system communication services.
Stream usage increases the memory storage space.
Streams make manipulating files in C++ much easier and safer.
Stream library of C++ allows for easier maintenance of the data.
Istream is used for formatted and unformatted input. Choose the WRONG statement:
Get() is used to extract a character
Getline() extracts character into an array or string
Read( ) extracts from unformatted data
Endfile () indicates end-of-file
Fstream class provides distinct classes for file processing. What does it NOT provide?
WRITE
READ
EXECUTE
None of the above
All of the above
It is a means for designing generic functions and generic classes.
Functions
Templates
Standard Template Library
Declaration
In order to define a function template, what keyword is used?
Template
Typename
Class
None of the above.
All of the above.
When is the template keyword properly used?
The template keyword is used before creating a function template or class template
The template keyword is used after creating a function template or class template
The function formal parameter type is passed using which method?
Pass by value
Pass by reference
Pass by assist
Pass by address
Which of the following is INCORRECT function template declaration?
Template < typename t >
Template { typename t }
Template < class t >
None of the above
All of the above
They perform identical operations for the different types of data compactly and conveniently. These are special functions that can operate with generic data types.
Templates
Function templates
Class Templates
Data templates
Function templates can be overloaded.
True
False
These are used where there are multiple copies of specific code for different data types with the same logic
Templates
Function Templates
Class templates
Data templates
Class templates can be inherited.
True
False
Template classes or functions generated from a template DO NOT have their own copies of any static variables or members.
True
False
Instantiations of function templates DO NOT have their own copies of any static variables defined within the scope of the function.
True
False
Class templates can be friends of:
A global function
A member function of another class (which can itself be a template class)
An entire class
All of the above
None of the above
Template code is not compiled until it is used (instantiated). This means the compiler WILL NOT catch syntax errors in a template until the template is used.
True
False
Templates provide a way to reuse _____ code, as opposed to inheritance and composition which provide a way to reuse _____ code.
Object; source
Source; object
Function; template
Template; function
Which of the following is FALSE about C++ Standard Template Library?
The Standard Template Library (STL) is a general-purpose C++ library of algorithms and data structures (It is based on a concept of generic programming, which is a part of the standard ANSI/ISO C++ library)
STL is implemented using template mechanisms and is a huge class library
STL is a C++ library of container classes, algorithms and iterators
It provides many of the basic algorithms and data structures of computer science
None of the above
All of the above
Which of the following is NOT a benefit of C++ Standard Template Library?
Consists of powerful template-based components and data structures (STL supports algorithms, data manipulation, searching, sorting, etc.)
Is a powerful and versatile collection of classes and functions
Provides an efficient, lightweight and extensible framework for application development
Aligns with one of the key benefits of object-oriented programming: reuse, reuse, reuse!
None of the above
All of the above
The Standard Template Library in C++ mainly consists of:
Container Classes
Iterators
Algorithms
All of the above
None of the above
Classes whose purpose is to contain other objects. They are templates for data structures.
Container Classes
Iterators
Algorithms
Class Templates
The mechanisms that makes it possible to decouple algorithms from containers. Consist of pointers and access elements for containers.
Container Classes
Iterators
Algorithms
Class Templates
Manipulate the data stored in containers. They support data manipulation, searching, sorting, etc.
Container Classes
Iterators
Algorithms
Class Templates
A container is an object that stores other objects and has methods for accessing its elements. Which of the following is NOT a type of container?
Sequence containers
Associative containers
Transitive containers
Container adapters
Included in the categories of algorithm are?
Non-modifying
Mutating-Copy
Sorting Related
Numeric-Accumulate
All of the above
None of the above
It provides applications with mid-level access to most database systems via Structured Query Language (SQL).
Network Connectivity
Database Connectivity
Peer-to-peer Connectivity
Internet Connectivity
In the Open Database Connectivity (ODBC) Architecture, this level performs processing and calls ODBC functions to submit SQL statements and retrieve results.
Application
Driver Manager
Driver
Data source
In the Open Database Connectivity (ODBC) Architecture, this level loads and unloads drivers on behalf of an application. Processes ODBC function calls or passes them to a driver.
Application
Driver Manager
Driver
Data source
In the Open Database Connectivity (ODBC) Architecture, this level processes ODBC function calls, submits SQL requests to a specific data source, and returns results to the application. If necessary, the driver modifies an application's request so that the request conforms to syntax supported by the associated DBMS.
Application
Driver Manager
Driver
Data source
In the Open Database Connectivity (ODBC) Architecture, this level consists of the data the user wants to access and its associated operating system, DBMS, and network platform (if any) used to access the DBMS.
Application
Driver Manager
Driver
Data source
They occur before the program compiles; include external files, define symbolic constants, macros, conditional compilation, conditional execution
Template
Function
Source Code
Preprocessors
It can convert an expression of a given type into another type.
Casting
Conversion
Overriding
Overloading
The new C++ casting operators are intended to provide a solution to the shortcomings of the old C-style casts by providing:
Improved syntax. Casts have a clear, concise, although somewhat cumbersome, syntax. This makes casts easier to understand, find and maintain.
Improved semantics. The intended meaning of a cast is no longer ambiguous. Knowing what the programmer intended the cast to do makes it possible for compilers to detect improper casting operations.
Type-safe conversions. Allow some casts to be performed safely at run-time. This will enable programmers to check whether or not a particular cast is successful.
All of the above
None of the above
This casting operator is used for conversion between types (Type checking at compile time; Standard conversions: void* to char*, int to float, etc.; Base class pointers to derived class pointers)
Dynamic_cast
Static_cast
Reinterpret_cast
Const_cast
This casting operator is used for polymorphic programming (Often used to downcast the base class pointer to the derived class pointer; used with virtual functions)
Dynamic_cast
Static_cast
Reinterpret_cast
Const_cast
This casting operator is used to cast away const or volatile (volatile keyword is a type qualifier used to declare that an object can be modified in the program by something such as the operating system, the hardware, or a concurrently executing thread ; Get rid of a variable's "const-ness")
Dynamic_cast
Static_cast
Reinterpret_cast
Const_cast
This casting oeprator is used for non-standard casts (i.e. One ponter to another) and cannot be used for standard casts (i.e., double to int).
Dynamic_cast
Static_cast
Reinterpret_cast
Const_cast
RTTI determines an object's type at run time. RTTI stands for?
Real-Time Type Identification
Run-Time Type Identification
Real-Time Type Identifier
Run-Time Type Identifier
RTTI returns the name of the object as?
C-style string
Unsigned integer
Character
Void
The comment block in a header file describes the purpose and maintenance history of the file.
True
False
The comment block in a source file describes the purpose and maintenance history of the file.
True
False
Local variables should be declared immediately after use.
True
False
Fields should be declared public as it does not protect the user of the class from changes in class implementation.
True
False
It is a common practice to separate the members of a class according to their scope (private, protected, public). It is the programmer’s choice as to which will come first.
True
False
The first lines of a method are usually devoted to checking the validity of all arguments. The idea is to fail as quickly as possible in the event of an error. This is particularly important for constructors.
True
False
Assigning values of more than one variable per line of code is recommended.
True
False
If a method returns an array which can be empty, do not allow it to return null. Instead, return a zero-length array.
True
False
In file organization, C++ source file can contain a class. When private classes are associated with a public class, they can be placed in the same source file as the public class.
True
False
{"name":"Knowledge Check 5 Reviewer", "url":"https://www.quiz-maker.com/QPREVIEW","txt":"Test your understanding of strings, templates, and the C++ programming language with our comprehensive quiz. Dive deep into the fundamental concepts and see how well you can apply your knowledge in a challenging 60-question format!Explore key functions and members associated with strings.Understand the intricacies of templates and their applications.Perfect for learners and developers seeking to enhance their skills.","img":"https:/images/course4.png"}