CSCI 201L MT Quizzes
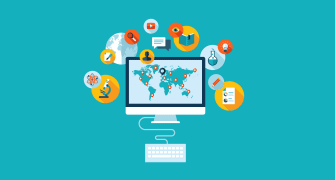
Java Programming Knowledge Quiz
Test your knowledge of Java programming concepts with this engaging quiz designed for both beginners and experienced developers. This quiz covers a variety of topics including object-oriented programming, concurrency, exception handling, and more!
Here’s what you can expect:
- 37 challenging questions
- Multiple-choice format
- Instant feedback on your answers
- Improve your Java skills
public class ThreadMaker {
public static void main (String[] args) {
System.out.print("First Line");
Thread ta = new Thread(new MyThread("a"));
Thread tb = new Thread(new MyThread("b"));
Thread tc = new Thread(new MyThread("c"));
ta.start();
tb.start();
tc.start();
try {
ta.join();
tb.join();
tc.join();
} catch (InterruptedException e) {
System.out.println("e: " + e.getMessage());
}
System.out.print("Last Line");
}
}
class MyThread implements Runnable{
String name;
MyThread(String name){
this.name = name;
}
public void run() {
for (int I = 0; I < 4; i++) {
System.out.print(name + I + " ");
}
}
}