PCMCQ-1
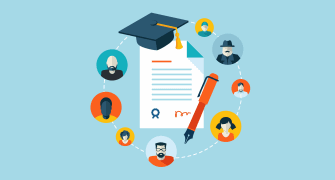
Mastering C and Data Structures Quiz
Test your knowledge and skills in C programming and data structures with our comprehensive quiz. With 29 carefully crafted questions, this quiz will challenge your understanding of arrays, linked lists, stacks, queues, and more!
Whether you're preparing for an exam or just want to brush up on your coding skills, this quiz offers:
- Multiple-choice questions
- In-depth concepts
- A mix of theory and practical scenarios
What is the Output of the following program?
int main()
{
int a[][] = {{1,2},{3,4}};
int I, j;
for (i = 0; I < 2; i++)
for (j = 0; j < 2; j++)
printf("%d ", a[i][j]);
return 0;
}
{
int a[][] = {{1,2},{3,4}};
int I, j;
for (i = 0; I < 2; i++)
for (j = 0; j < 2; j++)
printf("%d ", a[i][j]);
return 0;
}
1 2 3 4
Compiler Error in line ” int a[][] = {{1,2},{3,4}};”
4 garbage values
4 3 2 1
Consider the following C declaration.
char a[99][99];
What id the Address of a[10][10]?
(Size of the character is 1 byte)
char a[99][99];
What id the Address of a[10][10]?
(Size of the character is 1 byte)
901
900
899
902
What does the following declaration mean?
int (*ptr)[10];
Ptr is array of pointers to 10 integers
Ptr is a pointer to an array of 10 integers
Ptr is an array of 10 integers
Ptr is an pointer to array
In C, if you pass an array as an argument to a function, what actually gets passed?
Value of elements in array
First element of the array
Base address of the array
Address of the last element of array
What is the Output of the following code?
#include <stdio.h>
int main()
{
char p;
char buf[10] = {1, 2, 3, 4, 5, 6, 9, 8};
p = (buf + 1)[5];
printf("%dn", p);
return 0;
}
#include <stdio.h>
int main()
{
char p;
char buf[10] = {1, 2, 3, 4, 5, 6, 9, 8};
p = (buf + 1)[5];
printf("%dn", p);
return 0;
}
9
5
6
4
What is the Output of the following code?
#include <stdio.h>
int main()
{
int arri[] = {1, 2 ,3};
int *ptri = arri;
char arrc[] = {1, 2 ,3};
char *ptrc = arrc;
printf("sizeof arri[] = %d ", sizeof(arri));
printf("sizeof ptri = %d ", sizeof(ptri));
printf("sizeof arrc[] = %d ", sizeof(arrc));
printf("sizeof ptrc = %d ", sizeof(ptrc));
return 0;
}
#include <stdio.h>
int main()
{
int arri[] = {1, 2 ,3};
int *ptri = arri;
char arrc[] = {1, 2 ,3};
char *ptrc = arrc;
printf("sizeof arri[] = %d ", sizeof(arri));
printf("sizeof ptri = %d ", sizeof(ptri));
printf("sizeof arrc[] = %d ", sizeof(arrc));
printf("sizeof ptrc = %d ", sizeof(ptrc));
return 0;
}
12 4 3 4
3 4 3 4
12 3 12 3
4 3 4 3
Which of the following permutation can be obtained in the same order using a stack assuming that input is the sequence 5, 6, 7, 8, 9 in that order?
7 8 9 6 5
5 9 8 6 7
9 5 6 8 7
5 8 6 9 7
The best data structure to check whether an arithmetic expression has balanced parenthesis is
Queue
Stack
Linked List
Array
Which of the following is not an inherent application of stack?
Implementation of recursion
Evaluation of a postfix expression
Job scheduling
Reverse a string
What is the time complexity of pop() operation when the stack is implemented using an array?
O(1)
O(log N)
O(N^2)
O(N)
Output of the following code
int a[3] = {1,2,3};
*a = 100;
cout<<a[0];
Compilation error
100
Garbage value
1
Array is a
Pointer constant
Constant pointer
Pointer to a constant
Constant to a pointer
What does the following function do for a given Linked List with first node as head?
void fun1(struct node* head){
if(head == NULL) return;
fun1(head->next);
printf("%d ", head->data);}
Prints all nodes of linked lists
Prints all nodes of linked list in reverse order
Prints alternate nodes of Linked List
Prints alternate nodes in reverse order
What is the output of following function for start pointing to first node of following linked list? 1->2->3->4->5->6
void fun(struct node* start){
if(start == NULL) return;
printf("%d ", start->data);
if(start->next != NULL )
fun(start->next->next);
printf("%d ", start->data);}
1 4 6 6 4 1
1 3 5 1 3 5
1 2 3 5
1 3 5 5 3 1
In the worst case, the number of comparisons needed to search a singly linked list of length n for a given element is
Log 2 (n)
N/2
Log 2 (n – 1)
N
Suppose each set is represented as a linked list with elements in arbitrary order. Which of the operations among union, intersection, membership, cardinality will be the slowest?
Union only
Intersection, membership
Membership, cardinality
Union, intersection
What are the time complexities of finding 8th element from beginning and 8th element from end in a singly linked list? Let n be the number of nodes in linked list, you may assume that n > 8
O(1) and O(n)
O(1) and O(1)
O(n) and O(1)
O(n) and O(n)
Is it possible to create a doubly linked list using only one pointer with every node?
Not Possible
Yes, possible by storing XOR of addresses of previous and next nodes
Yes, possible by storing XOR of current node and next node
Yes, possible by storing XOR of current node and previous node
Given pointer to a node X in a singly linked list. Only one pointer is given, pointer to head node is not given, can we delete the node X from given linked list?
Possible if X is not last node. Use following two steps (a) Copy the data of next of X to X. (b) Delete next of X
Possible if size of linked list is even
Possible if size of linked list is odd
Possible if X is not first node. Use following two steps (a) Copy the data of next of X to X. (b) Delete next of X
You are given pointers to first and last nodes of a singly linked list, which of the following operations are dependent on the length of the linked list?
Delete the first element
Insert a new element as a first element
Delete the last element of the list
Add a new element at the end of the list
The concatenation of two lists is to be performed in O(1) time. Which of the following implementations of a list should be used?
Singly linked list
Doubly linked list
Circular doubly linked list
Array implementation of lists
Among the options select those which are the situations for using queue data structure
When a resource is shared among multiple consumers
When data is transferred asynchronously (data not necessarily received at same rate as sent) between two processes
Depth-First Search
Load Balancing
How many stacks are needed to implement a queue?
1
2
3
4
Which of the following is true about linked list implementation of queue?
In push operation, if new nodes are inserted at the beginning of linked list, then in pop operation, nodes must be removed from end
In push operation, if new nodes are inserted at the end, then in pop operation, nodes must be removed from the beginning
Both of the above
None of the above
A Priority-Queue is implemented as a Max-Heap. Initially, it has 5 elements. The level-order traversal of the heap is given below: 10, 8, 5, 3, 2 Two new elements ”1‘ and ”7‘ are inserted in the heap in that order. The level-order traversal of the heap after the insertion of the elements is:
10, 8, 7, 5, 3, 2, 1
10, 8, 7, 2, 3, 1, 5
10, 8, 7, 1, 2, 3, 5
10, 8, 7, 3, 2, 1, 5
Consider the following operation along with Enqueue and Dequeue operations on queues, where k is a global parameter
MultiDequeue(Q){
m = k
while (Q is not empty and m > 0)
{dequeue(q) m = m - 1}
}
What is the worst case time complexity of a sequence of n MultiDequeue() operations on an initially empty queue?
Theta(n)
Theta(n+k)
Theta(nk)
Theta(n^2)
A queue is implemented using an array such that ENQUEUE and DEQUEUE operations are performed efficiently. Which one of the following statements is CORRECT (n refers to the number of items in the queue)?
Both operations can be performed in O(1) time
At most one operation can be performed in O(1) time but the worst case time for the other operation will be Ω(n)
The worst case time complexity for both operations will be Ω(n)
Worst case time complexity for both operations will be Ω(log n)
Consider the following pseudo code. Assume that IntQueue is an integer queue. What does the function fun do?
void fun(int n){
IntQueue q = new IntQueue();
q.enqueue(0);
q.enqueue(1);
for (int I = 0; I < n; i++) {
int a = q.dequeue();
int b = q.dequeue();
q.enqueue(b);
q.enqueue(a + b); ptint(a);
}
}
Prints numbers from 0 to n-1
Prints numbers from n-1 to 0
Prints first n Fibonacci numbers
Prints first n Fibonacci numbers in reverse order
A normal queue, if implemented using array of size MAX_SIZE, gets full when
Rear=MAX_SIZE-1
Rear=front
Front=rear+1
Front=(rear+1)%MAX_SIZE
{"name":"PCMCQ-1", "url":"https://www.quiz-maker.com/QPREVIEW","txt":"Test your knowledge and skills in C programming and data structures with our comprehensive quiz. With 29 carefully crafted questions, this quiz will challenge your understanding of arrays, linked lists, stacks, queues, and more!Whether you're preparing for an exam or just want to brush up on your coding skills, this quiz offers:Multiple-choice questionsIn-depth conceptsA mix of theory and practical scenarios","img":"https:/images/course1.png"}