Data Structures Quiz
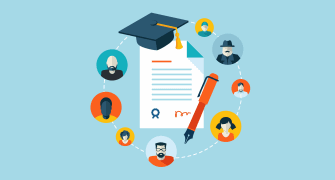
Data Structures Quiz
Test your knowledge of data structures with our comprehensive quiz! This quiz features 40 carefully crafted questions covering various essential topics, guaranteeing a robust understanding of data structures.
Whether you are a student, educator, or just a data structure enthusiast, this quiz is for you. Highlights include:
- Multiple choice questions
- Covers linked lists, arrays, stacks, and more
- Instant feedback on your answers
What will be the output of the following code snippet for the list 1->2->3->4->5->6? Void solve(struct node* start) { if(start == NULL) return; printf("%d ", start->data); if(start->next != NULL ) solve(start->next->next); printf("%d ", start->data); }
1 2 3 4 5 6
1 3 5 5 3 1
1 3 5 1 3 5
2 4 6 1 3 5
What does the following code snippet do? ListNode* solve(ListNode* head) { ListNode* prev = NULL; if(head == NULL) { return head; } if(head -> next == NULL) { return head; } ListNode* curr = head -> next; while(head != NULL) { head -> next = prev; prev = head; head = curr; if(curr != NULL) { curr = curr -> next; } } return prev; }
Returns the original linked list
Returns the linked list after reversing it
Returns a linked list containing elements at odd indices only
None of these
Which of the following algorithm is the optimal way to find the middle element of the linked list?
Find length, and then traverse to length / 2 th node.
Fast and slow pointer method
Find distance of all nodes, and print middle nodes.
None
Which of the following application makes use of a circular linked list?
Undo operation in a text editor
Recursive function calls
Allocating CPU to resources
Implement Hash Tables
Which of the following is false about a circular linked list?
Every node has a successor
Time complexity of inserting a new node at the head of the list is O(1)
Time complexity for deleting the last node is O(n)
We can traverse the whole circular linked list by starting from any point
We can traverse the whole circular linked list by starting from any point
piling up of chairs one above the other
People standing in a line to be serviced at a counter
Offer services based on the priority of the customer
Tatkal Ticket Booking in IRCTC
.What is the time complexity of pop() operation when the stack is implemented using an array?
O(1)
O(n)
O(logn)
O(nlogn)
Which of the following array position will be occupied by a new element being pushed for a stack of size N elements(capacity of stack > N)?
S[n-1]
S[1]
S[n]
S[0]
Which of the following concepts make extensive use of arrays?
Binary trees
Scheduling of processes
Caching
Spatial locality
.Assuming int is of 4bytes, what is the size of int arr[15];?
15
19
11
60
Infix to postfix expression. ((a/(b-c+d))*(e-a)*c)
Ab-cd/+e
abc-d+/ea-c
abcd-+e/a*-c*
abc-d+e/a-c
Nfix to postfix expression. a/b-c+d*e-a*c
ab/c-de*+ac*-
Abc/-d*e+a*c-
Ab/cd-e*+a*c-
abc/d-e+a*c*-
Infix to prefix expression. a/b-c+d*e-a*c
-+-a/bc*de*ac
-+a-b/cd*e*ac
-+-/abc*de*ac
-+-/ab/cd*ea*c
Infix to prefix expression. a*(b+c)/d-g
-/a*bc+dg
. -/*ab+cdg
-/ab*cd+g
. -/*a+bcdg
Solve the postfix expression using stack operations. ab/c-db*+ a=6 b=2 c=3 d=4
10
8
16
None
.A LL in which insertion and deletion are made to from either ends of the structure
Circular queue
Random of queue
Dequeue
Priority
.A linked list node can be implemented/accessed using? a)Structs b)Class c)pointers d)arrow characters
A &b
A,b&d
All
A,b&c
.The disadvantage in using a circular linked list is
.last node points go the first node
Requires more memory spaces
It is possible to get into infinity loop
Time consumption
Node.next -> node.next.next; will make
Node.next inaccessible
Node.next.next inaccessible
This node inaccessible
None
Which of the following algorithms is not feasible to implement in a linked list?
Linear search
Linear sorting
Binary sorting
Binary search
How is the 2nd element in an array accessed based on pointer notation?
*a + 2
*(a + 2)
*(*a + 2)
&(a + 2)
From following which is not the operation of data structure?
A) Operations that manipulate data in some way
Operations that perform a computation
A) Operations that manipulate data in some way b Operations that check for syntax errors
A) Operations that manipulate data in some way b) Oper Operations that monitor an object for the occurrence of a controlling event
What will be the output of the following code snippet? Void solve() { int a[] = {1, 2, 3, 4, 5}; int sum = 0; for(int I = 0; I < 5; i++) { if(i % 2 == 0) { sum += a[i]; } } cout << sum << endl; }
5
15
9
6
Which of the following is a linear data structure?
Arrays
Trees
Binary trees
Graphs
Which data structure is mainly used for implementing the recursive algorithm?
Queue
Stack
Array
LL
What will be the output of the following C program? #include int main() { int x[5] = { 10, 20, 30 }; printf("%d", x[-1]); return 0; }
0
10
Garbage
None
What will be the output of the following C code? #include int main() { char str1[] = "Hello"; char str2[10]; str2 = str1; printf("%s,%s", str1, str2); return 0; }
Hello
Hello hello
Hello hello hello
Error
What is the output of this program? #include #include int main() { int numbers = (int)calloc(4, sizeof(int)); numbers[0] = 2; free(numbers); printf("Stored integers are "); printf("numbers[%d] = %d ", 0, numbers[0]); return 0; }
Garbage
2
0
Compilation error
. What will be the output of the program ? #include int main() { int a[5] = {5, 1, 15, 20, 25}; int I, j, m; I = ++a[1]; j = a[1]++; m = a[i++]; printf("%d, %d, %d", I, j, m); return 0; }
2 1 5
1 2 5
3 2 15
None
Consider the following C program segment: char p[20]; char *s = "string"; int length = strlen(s); int I; for (i = 0; I < length; i++) p[i] = s[length — i]; printf("%s", p); The output of the program is?
Gnirt
Gnirts
String
No output
In a DLL the no. Pointers affected for an insertion operation will be
4
8
1
Depends on node
Which of the following is false about the DLL
We can navigate in both the directions
It requires more space then the SLL
Operations takes longer time periods
None
What will be the output for the following code for list1->2->3->4->5->6! { if(start==NULL;return;printf("%d",start->data);if(start->next!=NULL)solve(start->next->next;printf("%d",start->data);}
1 2 3 4 5 6
1 3 5 5 3 1
1 3 5 1 3 5
2 4 6 1 3 5
How do you calculate the pointer difference in a memory efficient DLL?
Head xor tail
Pointer to prev node to pointer to next node
*prev node->*next node
*next node->*prev node
DLL contains how many fields
1
2
3
All of the above
How many stacks are needed to implement a queue .consider the situation where no other data structures are used
1
2
3
4
A priority queue can efficiently implemented using which of the following data structures?
Array
LL
Heap data structures
None
A priority queue is implemented as a max-heap. Intially it has 5 elements . The level-order traversal of the heap is given below:10 9 5 3 2 two new elements are added '1','7' into the heap in that order.the level-order traversal of the heap after the insertion of the elements is
10 8 7 5 3 2 1
10 8 7 2 3 1 5
10 8 7 1 2 3 5
10 8 7 3 2 1 5
Type of queue in which the operations can take place from both directions
Circular queue
Double ended queue
Circular double ended queue
None
If the inserted elements are in the queue are1,2,3,4 and it's max value is 4 does insertion of another element take place
Yes
No
Using deletion once and then inserting
B &c
{"name":"Data Structures Quiz", "url":"https://www.quiz-maker.com/QPREVIEW","txt":"Test your knowledge of data structures with our comprehensive quiz! This quiz features 40 carefully crafted questions covering various essential topics, guaranteeing a robust understanding of data structures.Whether you are a student, educator, or just a data structure enthusiast, this quiz is for you. Highlights include:Multiple choice questionsCovers linked lists, arrays, stacks, and moreInstant feedback on your answers","img":"https:/images/course1.png"}