Searching and Sorting Algorithms
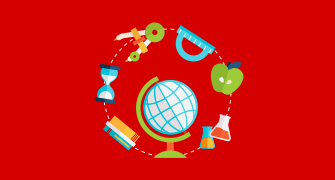
Mastering Searching and Sorting Algorithms
Test your knowledge of essential searching and sorting algorithms with this engaging quiz! Cover a variety of topics including binary search, quick sort, and much more.
In this quiz, you will learn:
- Key concepts in searching algorithms
- Differences between sorting methods
- Practical application of algorithms in programming
Which of the following is not a limitation of binary search algorithm?
Must use a sorted array
There must be a mechanism to access middle element directly
requirement of sorted array is expensive when a lot of insertion and deletions are needed
binary search algorithm is not efficient when the data elements more than 1500.
The worst-case occur in linear search algorithm when
Item is somewhere in the middle of the array
. Item is not in the array at all
Item is the last element in the array
Item is the last element in the array or item is not there at all
The Average case occurs in the linear search algorithm
When the item is the last element in the array
When the item is somewhere in the middle of the array
When the item is not the array at all
Item is the last element in the array or item is not there at all
Which of the following sorting algorithm is of divide and conquer type?
Bubble sort
Insertion sort
Merge sort
Selection sort
Partition and exchange sort is
quick sort
Heap sort
bubble sort
Mearge Sort
Which of the following algorithm does not divide the list
Linear search
Binary search
Merge sort
Quick sort
Quick sort algorithm is an example of
Greedy approach
Improved binary search
Dynamic Programming
Divide and conquer
Quick sort running time depends on the selection of
Size of array
Pivot element
Sequence of values
None of the above
How many swaps are required to sort the given array using bubble sort - { 2, 5, 1, 3, 4}
4
5
6
7
The worst case complexity of binary search matches with
Interpolation search
Linear search
Merge sort
None of the above
State True or False.
i) Binary search is used for searching in a sorted array.
ii) The time complexity of binary search is O(logn).
True
False
A technique for direct search is
Binary Search
Linear Search
Linear Search
Hashing
Which of the following is not an in-place sorting algorithm?
Bubble Sort
Heap Sort
Quick Sort
Merge Sort
A sorting technique is called stable if it
Takes O(n log n) times
Maintains the relative order of occurrence of non-distinct elements
Uses divide-and-conquer paradigm
Takes O(n) space
Select the appropriate code that performs bubble sort.
for(int j=arr.length-1; j>=0; j--) {
for(int k=0; k<j; k++) {
if(arr[k] > arr[k+1]) {
int temp = arr[k];
arr[k] = arr[k+1];
arr[k+1] = temp;
}
}
}
for(int j=arr.length-1; j>=0; j--) { for(int k=0; k<j; k++) { if(arr[k] < arr[k+1]) { int temp = arr[k]; arr[k] = arr[k+1]; arr[k+1] = temp; } } }
for(int j=arr.length; j>=0; j--) { for(int k=0; k<j; k++) { if(arr[k] > arr[k+1]) { int temp = arr[k]; arr[k] = arr[k+1]; arr[k+1] = temp; } } }
for(int j=arr.length; j>=0; j--) { for(int k=0; k<j; k++) { if(arr[k] > arr[k+2]) { int temp = arr[k]; arr[k] = arr[k+1]; arr[k+1] = temp; } } }
{"name":"Searching and Sorting Algorithms", "url":"https://www.quiz-maker.com/QPREVIEW","txt":"Test your knowledge of essential searching and sorting algorithms with this engaging quiz! Cover a variety of topics including binary search, quick sort, and much more.In this quiz, you will learn:Key concepts in searching algorithmsDifferences between sorting methodsPractical application of algorithms in programming","img":"https:/images/course8.png"}
More Quizzes
Binary Search Algorithm
420
Sorting Algorithm
740
5.Asymptotic Notations and Basic Efficiency Classes
4266
Data structure Quiz
10516
Notion of an Algorithm, Importance & Role of algorithms in computing
5272
XII-COMP REVISION TEST
10510
Big O(Notation)
7459
Technical Round
15819
CS101 Midterm Training
17844
Cc103 lolzki 2
1059
DSA LAB ASSESSMENT
10537
Binary
12611