Karel Coding Challenge
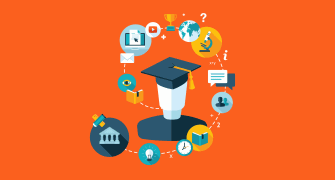
Karel Coding Challenge
Welcome to the Karel Coding Challenge! Test your knowledge of programming concepts and Karel commands with our engaging quiz.
Challenge yourself and learn more about:
- Karel's movements and commands
- JavaScript fundamentals
- Effective programming practices
Which of the following commands is a valid Karel command?
Move
Move;
Move();
Move()
Karel starts at Street 1 and Avenue 1, facing East. After calling the stairStep function twice, where will Karel be and what direction will Karel be facing? (assume this is a SuperKarel program and the world is 10x10 in size)
function stairStep() {
move();
turnLeft();
move();
turnRight();
}
Street 2, Avenue 2, Facing North
Street 3, Avenue 3, Facing East
Street 3, Avenue 3, Facing West
Street 4, Avenue 4, Facing East
What’s wrong with this code?
function start() {
move();
go();
go();
}
function go() {
move();
move();
}
function go() {
move();
move();
}
The go fun ction is called twice
The go function has a syntax error
The go fun ction has been defined twice
Go is not a command that Karel understands
What does the mystery function do?
function mystery() {
while (noBallsPresent()) {
move();
}
}
Karel moves until it is on a ball
Karel moves once if there is no ball present
Karel moves until it puts down a ball
Karel checks if there is no ball on the current spot and then moves once
Why does a programmer indent their code?
Helps show the structure of the code.
Easier for other people to understand.
A key part of good programming style!
All of the above
How can we teach Karel new commands?
For loop
While loop
Define a new function
The start function
What is the purpose of using a for loop in code?
To do something if a condition is true
To do something while a condition is true
To repeat something a fixed number of times
To make programs run faster
A store has 20 apples in its inventory. How can you store this information in a JavaScript variable?
Var numApples == 20;
20 = numApples;
var numApples = 20;
Var num apples = 20;
You are splitting up all of your apples equally between 3 people. Which statement below will calculate how many apples will be left over?
var leftOver = numApples / 3;
var leftOver = 3 / numApples;
var leftOver = numApples % 3;
var leftOver = 3 % numApples;
What is the output of the following program: (Assume the user enters ‘Florence’ then ‘Fernandez’.)
var first_name = readLine("What is your first name? ");
var last_name = readLine("What is your last name? ");
var whole_name = first_name + last_name;
println (whole_name);
Fernandez Florence
Florence
Fernandez
Fernandez
FlorenceFernandez
Florence Fernandez
If you were given the following variables:
var distance = 2;
var time = 30;
What line of code would print the speed in km/hr if the distance is given in km and the time is given in minutes? (Speed = distance/time)
Println(distance/(time/60));
Println(distance/time);
Println(distance*(time*60));
Println(distance*time);
What is printed to the screen when the following program is run?
var num = 13 % 3;
println(num);
1
2
3
4
If a user enters a diameter value in the variable diameter, how would we use this to draw the correct sized circle?
var circle = new Circle(diameter);
circle.setPosition(100,100);
add(circle);
var circle = new Circle(diameter*2);
circle.setPosition(100,100);
add(circle);
var circle = new Circle(diameter/2);
circle.setPosition(100,100);
add(circle);
var circle = new Circle(diameter%2);
circle.setPosition(100,100);
add(circle);
What is printed to the screen if the user enters ‘5’ when the following program is run?
var userNum = readInt("Enter your favorite number: ");
var calculatedNum = (userNum * userNum) + userNum;
println(calculatedNum);
5
25
30
50
Which of the following choices is a properly formed JavaScript variable name, meaning it is both legal in the JavaScript language and considered good style?
user_age
UserAge
userAge
User age
What is the proper format for a single line comment in Karel?
This is a comment
// This is a comment
/* This is a comment
This is a comment
What condition should be used in this while loop to get Karel to pick up all the tennis balls on the current location?
while (________) {
takeBall();
}
NoBallsPresent()
BallsPresent()
FrontIsClear()
takeBall()
In a graphics canvas, what are the coordinates of the bottom right corner of the window?
0, 0
0, getWidth()
GetHeight(), getWidth()
getWidth(), getHeight()
What is the best way for Karel to move 10 times?
move();
move();
move();
move();
move();
move();
move();
move();
move();
move();
for (var I = 0; I < 10; i++) {
move();
}
Move(10);
Move10();
Which fun
A
function spin() {
turnRight();
}
B
function spin() {
turnLeft();
turnLeft();
turnLeft();
turnLeft();
}
C
function spin() {
turnLeft();
turnLeft();
}
D
function spin() {
move();
move();
move();
move();
}
A
B
C
D
Why do we use while loops in JavaScript?
To break out of some block of code
To do something if a condition is true
To repeat some code while a condition is true
To repeat something for a fixed number of times
Which general while
loop definition is written correctly?
A
while (x is true) {
// code
}
B
if (i<5) {
//code
}
C
while (var I = 0; I < count; i++) {
//code
}
D
while (condition) {
//code
}
A
B
C
D
What is the role of the driver in a pair-programming setting?
They keep track of the big picture
They tell the navigator what to do
They control the mouse and keyboard
They alert the navigator of typos and errors
Variables are essentially?
Place Holders for other stuff
Name for something else
Stuff
CSS
A special form of HTML
In the following image, what is the variable?
EyeSize
Eye Size
NoStroke
Var
Variable
In the following image, what is the given "value" for the only "variable?
20
0
255
300
65
If you were to change the "eyeSize" value of 28 to any number, would the code on lines 13 and 14 be affected?
Yes they would!
Nope, nothing would happen to the code.
Can the command "fill" affect the coloring of a "line" command?
Yes, it can.
No, it can't.
May be
In the following image, which code most likely corresponds to where the arrow is pointed at?
StrokeWeight (33);
line(9,3,100);
Ellipse (25,100,66);
Square(200,50,100);
Fill (255,0,0);
The 3 parameters used for coloring in JavaScript use what abbreviations?
R, G, B
C, Y, M, K
A, B, C
X, Y, Z
What type of quotation marks does JavaScript require?
Single quotes (aka apostrophes)
Double quotes
Either single quotes or double quotes
Neither single quotes or double quotes
What character do we use for multiplication?
#
/
*
X
What character do we use for division?
#
/
*
X
How do we count the number of characters in a string?
.length
.numberOfCharacters
.textLength()
String.characters()
A piece of code that combines commands and that you can easily call over and over again.
A function
A variable
A loop
A parameter
What function do you need to call to ask the user of the program to enter text?
ReadLine
Readln
Text
Println
To ask the user of the program for a number, which function should you use?
ReadLine
ReadInt
ReadFloat
Both readInt and readFloat are for numbers.
What is the proper function to call to print to the screen?
Println
Write
PRINT
Post
How do we convert a string to all caps?
.allCaps
.AllCaps()
.allCaps()
.toUpperCase()
Do you need to first define your variable before using it? Or can you use your variable first and then define it?
No, you can first use your variable and later define it.
Yes, you need to first define your variable
{"name":"Karel Coding Challenge", "url":"https://www.quiz-maker.com/QPREVIEW","txt":"Welcome to the Karel Coding Challenge! Test your knowledge of programming concepts and Karel commands with our engaging quiz.Challenge yourself and learn more about:Karel's movements and commandsJavaScript fundamentalsEffective programming practices","img":"https:/images/course3.png"}