Principles of Programming
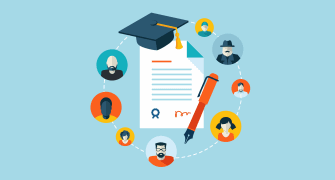
Test Your Programming Principles Knowledge!
Welcome to our Principles of Programming quiz! This engaging quiz is designed to test your understanding of fundamental programming concepts, terminology, and best practices.
Whether you are a student looking to reinforce your coding knowledge or a teacher seeking a fun way to assess your students, this quiz offers:
- 25 thought-provoking questions
- A mix of multiple-choice and checkbox answers
- A time-efficient way to gauge your programming prowess
What is the value of ‘number’ after the following code fragment execution?
public static void main(String[] args) {
int number = 0;
int number2 = 12;
while (number < number2)
{
number = number + 1;
System.out.println(number);
}
}
Select one:
Which of the following options is the correct declaration of an integer type variable called numberOfMonths to which the value of 6 is assigned?
Select one or more:
Which of the following statements are accurate?
Select one or more:
What is an assignment statement?
Select one:
What is the correct declaration statement for a simple integer array of 6 elements?
*The actual correct answer is 'd', but the quiz says that 'c' is correct, which it isn't*
Which of the following are methods associated with the String class?
A). length()
B). toLowerCase()
C). equals()
D). to UpperCase()
Select one:
Which of the following statements are true? Tick all that apply.
Select one or more:
Why is String declared using a capital S in Java?
Select one:
Which of the following is considered as a blue print that defines the variables and methods common to all of its objects of a specific kind?
- Object
B. Class
C. Method
D. Real data types
Select one:
Which is a valid declarations of a String?
- String s1 = null;
- String s2 = 'null';
- String s3 = (String) 'abc';
- String s4 = (String) '\ufeed';
Select one:
What is the correct declaration of a variable to hold a person's name?
Select one:
Which three are legal array declarations?
- int [] myScores [];
- char [] myChars;
- int [6] myScores;
- Dog myDogs [];
- Dog myDogs [7];
Select one:
Which of these is not a feature of all loops?
Select one:
In a String type array (names) holding the names of students in a class, what will the following statement produce?
Catherine |
Alexandra |
Daniel |
David |
Angela |
Simon |
System.out.println(names.1);
Select one:
What is a loop?
Select one:
Which of the following statements is true?
Select one or more:
Which of the following statements about the Java language is true?
- Both procedural and OOP are supported in Java.
- Java supports only procedural approach towards programming.
- Java supports only OOP approach.
- None of the above.
Select one:
Algorithmic thinking is the process of....
Select one:
What will be the output of the program?
class Test
{
public static void main(String [] args)
{
Test p = new Test();
p.start();
}
void start()
{
boolean b1 = false;
boolean b2 = fix(b1);
System.out.println(b1 + " " + b2);
}
boolean fix(boolean b1)
{
b1 = true;
return b1;
}
}
Select one: